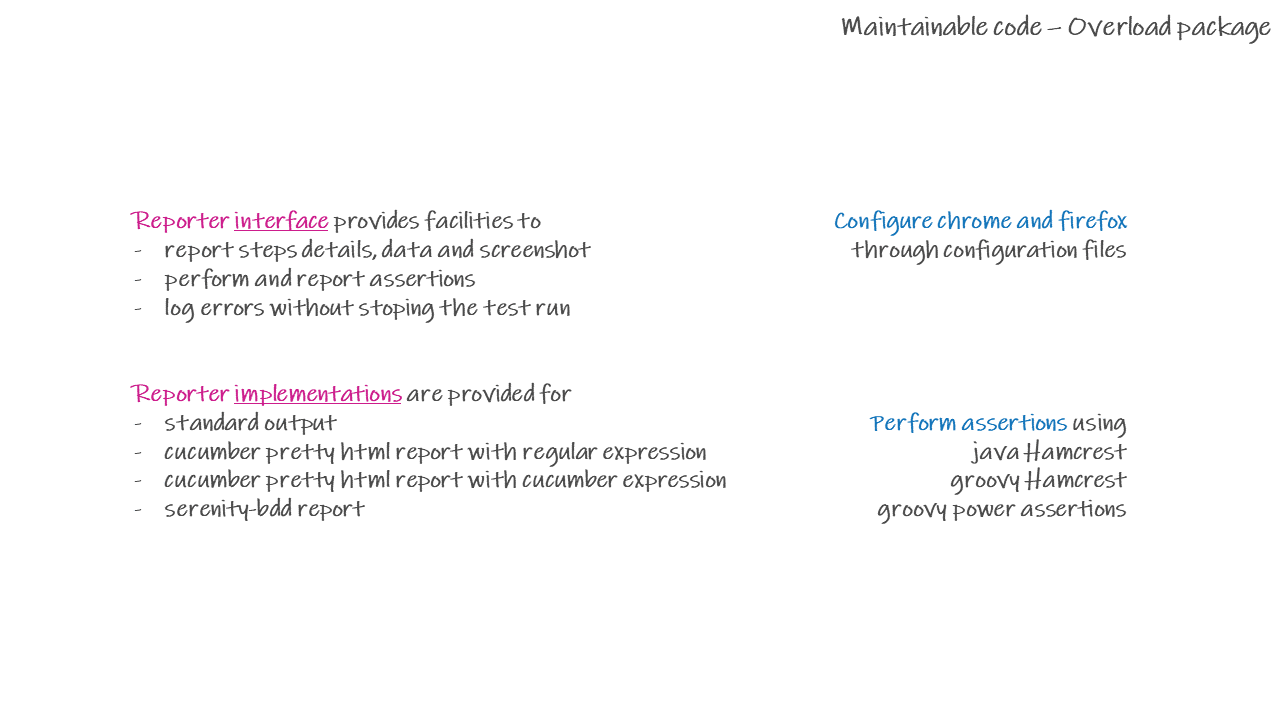
Main purpose
WebDriver Overload is a set of libraries to support automation engineers when applying Simple Methodology. It can be used out of the box or customized with your own implementation.
WebDriver Overload pagckage provides facilities to- WebDriver & Options providers
- Report test details
- Perform (none blocking) checks
- Integrate with other frameworks
Versions
- 2.0.0 including new Reliable elements for Reliable actions
WebDriver & Options providers
DriverProvider
A simple WebDriver provider for chrome (default) and firefox supporting local and grid.
For local WebDrivers [static WebDriver get(String browser, Path driverPath, String optionsAsYamlResource)] the parameters are
- String browser : Firefox or chrome, if other then it will be chrome (ex: "firefox")
- Path driverPath : Path of the excutable file (ie: Paths.get("C:/dev_tools/webdriver/geckodriver.exe"))
- String optionsAsYamlResource : Name of the resource file describing browser's options
For selenium grid [static WebDriver get(String browser, URL seleniumServer, String optionsAsYamlResource)] the parameters are
- String browser : Firefox or chrome, if other then it will be chrome (ex: "firefox")
- URL seleniumServer : the url of the selenium grid (ie: new URL("http://127.0.0.1:4444"))
- String optionsAsYamlResource : Name of the resource file describing browser's options
ChromeOptionsProvider
The static get function returns a ChromeOptions object based on options given in parameter.
ChromeOptionsProvider supports binary location, arguments, capabilities and extensions.
- static ChromeOptions get() will return default Chrome options
- static ChromeOptions get(String optionsAsYamlResource) will return Chrome options based on what is specified in the yaml file
FirefoxOptionsProvider
The static get function returns a ChromeOptions object based on options given in parameter.
FirefoxOptionsProvider supports binary location, arguments, capabilities, preferences and profile preferences and extensions.
- static FirefoxOptions get() will return default Firefox options
- static FirefoxOptions get(String optionsAsYamlResource) will return Firefox options based on what is specified in the yaml file
Available configuration
- "chrome_options_default.yml" will be used as default for Chrome
- "chrome_options_headless.yml" can be used for Chrome in headless mode
- "firefox_options_default.yml" will be used as default for Firefox
- "firefox_options_headless.yml" can be used for Firefox in headless mode
Custom configuration
You can provide your own configuration (ex : 'my_chrome_options'). Just copy/paste one of the existing configuration and update it as you wish. To get a driver with your configuration, use WebDriver.get("chrome", "C:/dev_tools/webdriver/chromedriver.exe", "my_chrome_options.yml").
Report test details
We believe the reporting is one of the key success factor for test automation.
There are two types of test details, everything from ghekin and the rest. There are several cucumber reporters available and we recommend to use your favorite reporter for all information at gherkin level. By reporting test details we mean showing additionnal usefull data about what is happening within a bdd step. Basically it is about test steps, test data, test evidence and screenshots. all these information is in addition to the standard cucumber reporters.
WebDriver Overload commes with an abstract layer of the reporting to define common reporting functions as well as a reporting implementation.
The Reporter, an abstract layer
The Reporter is an Interface object including default behaviour.
- Errors book : Functions used to collect and report deviation detected during test executions
- Reporting capabilities : List of reporting functions
- Checks : Default checks implementation combining assertions, logging errors and sending everything to the reporter
Errors book
This list of errors allow to log deviation while executing the tests without throwing exception and stopping the test.
Available functions are :
- clearErrors() : removes all errors in the book
- hasErrors() : returns true if there are errors in the book
- getErrorsSummary() : returns the all content of the book as a String
- throwAssertionErrorIfAny(boolean reportErrorsSummary) : if they are errors in the book an AssertionError is thrown and the test stops
Errors are added to the book using the reportError() functions.
Reporting
Here under kind of reporting capabilities. Implemention will depends on the selected reporter.
- reportAction(String action) : Report an action that represents what the automaton (as a user of the application) is doing in the application (ex: create an account)
- reportData(String or Path data) : Report the data used when doing the action
- reportCheck(String check) : Report a check that represents what we (as a tester) are verifying. It includes a screenshot.
- reportError(String or Path error) : Report the error description that represents the mismatch between the actual and the expected value. It includes adding the error in the errors book.
Reporter implementations
SystemOutReporter, usefull logger
This reporter will report your desired information in the system out console. It can print every kind of data like test steps, test data, deviations, error book. There is nothing about printscreens.
Concrete implementation in ohrm-tests github repository, branch 'cucumber-systemout'.
SerenityReporter, all in one reporter
Serenity reporter includes everything you need ... and much more. In addition to standard gherkin information Serenity reporter provides capabilities to add step informations, step data, screenshots, tags, requirements, capabilities, ...
Concrete implementation in ohrm-tests github repository, branch 'main'.
Perform (none blocking) checks
Checks and none blocking checks?
Unit test best practices says we should check only one thing per test. Despite this is a good best practice in unit testing, is it a good best practice for higher levels like UI or end-to-end automated test? ... If we are talking about test objectif, the answer should definitively yes, one test has a unique test objective. Now if we think about checks the answer may be different. Lets take the case were the test objective is to verify that a user can create an account. To do that, the user will navigate throught few pages andthe tester want to check if the user can create an account and use these opportunity to check the title of the account creation page. The question is, is it efficient to write 2 test cases, one to check the title of the page and one to check the account creation?
At simple4tests we think it is convenient, in this case, to write one test to check the account creation within which we check the title of the page. One of the conditions to apply this kind of rules is to have a simple and clean way of reporting what happens in the test. It is why, checks and reporting are related concept in webdriver-overload package. In practice Webdriver-Overload provide checks functions through the Reporter object. Details on reporting can be found a little lower.
How checks are performed using webDriver-Overload
Checks are made of 3 information, the name of the check, the actual value, and the expected value or expression.
It consists of the following steps
- Add a new step in the report using the name of the check
- Compares the actual value with the expected expression
- In case of match, continue running the test
- In case of no match, reports the deviation as evidence or test data, logs the deviation in an error book for futhur analyse and continue running the test
Checks using Java Hamcrest Matchers
reporter.assertThat("Check page title", Automaton.getPageTitle(), Matchers.equalTo("Account creation"));
This function allow to describe expected value as Hamcrest Matchers expression.
Some exemples
- Matchers.equalTo(...)
- Matchers.containsStringIgnoringCase(...)
- Matchers.greaterThan(...)
Hamcrest Matchers support String, Integer, List, Object, ... A lot of documentation si available on internet.
Checks using Groovy Hamcrest Matchers
reporter.groovyAssertThat("Check page title", Automaton.getPageTitle(), "equalTo('Account creation')");
This function allow to describe expected value as Hamcrest Matchers expression. The difference is, in this way the description is not a java function but a litteral. We can now describe checks on external files as String.
To achieve it WebDriver Overload uses a groovy engine to evaluate the expression using 'assertThat' command.
Checks using Groovy power assertions
reporter.groovyAssert("Check page title", String.format("'%s'.equals('Account creation')", Automaton.getPageTitle()));
This function allow to describe any groovy assert expression. Previously we used an object and an expression to compared with. Now we use a sinple expression.
To achieve it WebDriver Overload uses a groovy engine to evaluate the expression using 'assert' command.
Integrate with other frameworks
Serenity BDD
We choose to provide the necessary tools to integrate with serenity-bdd because we found its reporter very complete.
The integration is about
- SerenityDriver used to start the Serenity engine
- serenityReporter that implements the WedDriver Overload Reporter
Concrete implementation in ohrm-tests github repository, branch 'main'.