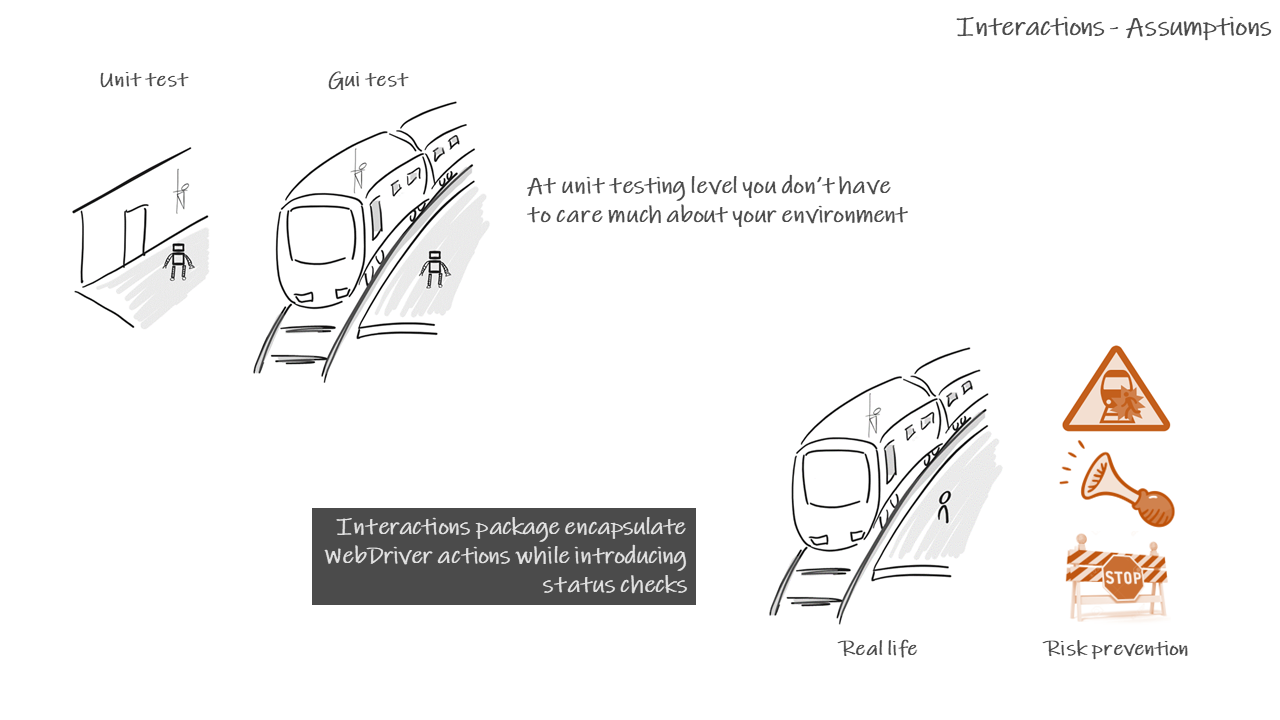
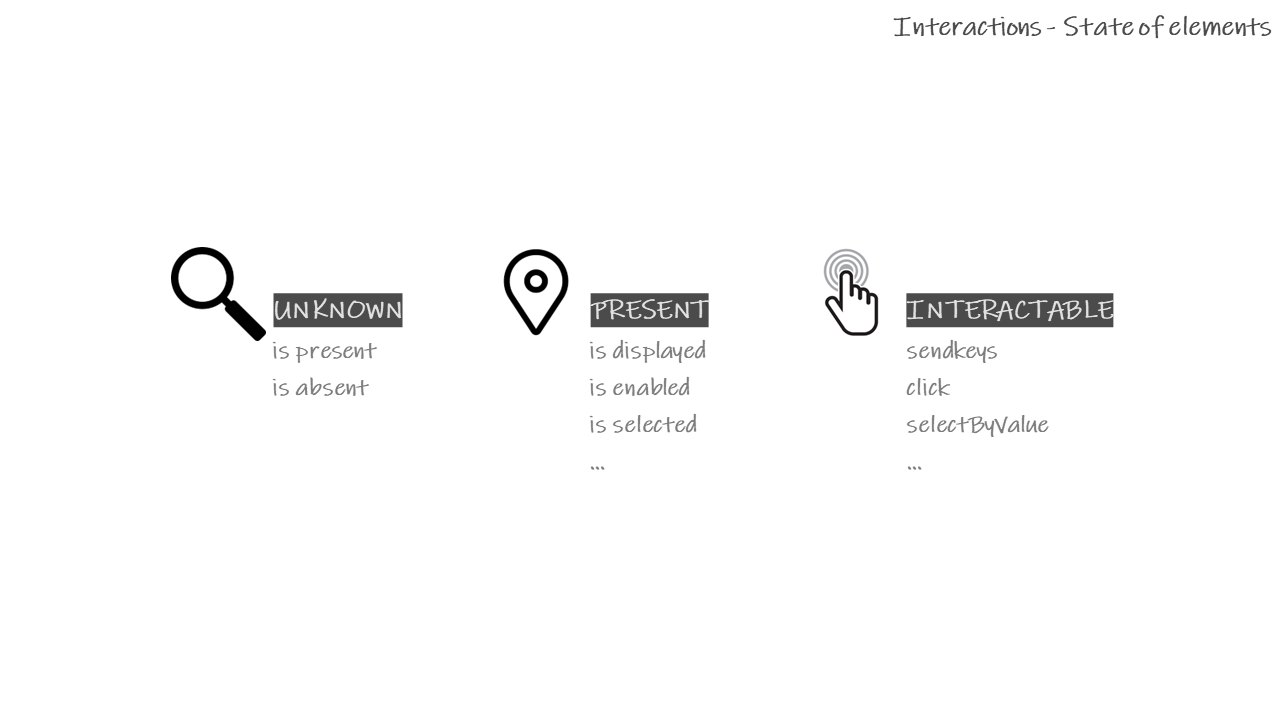
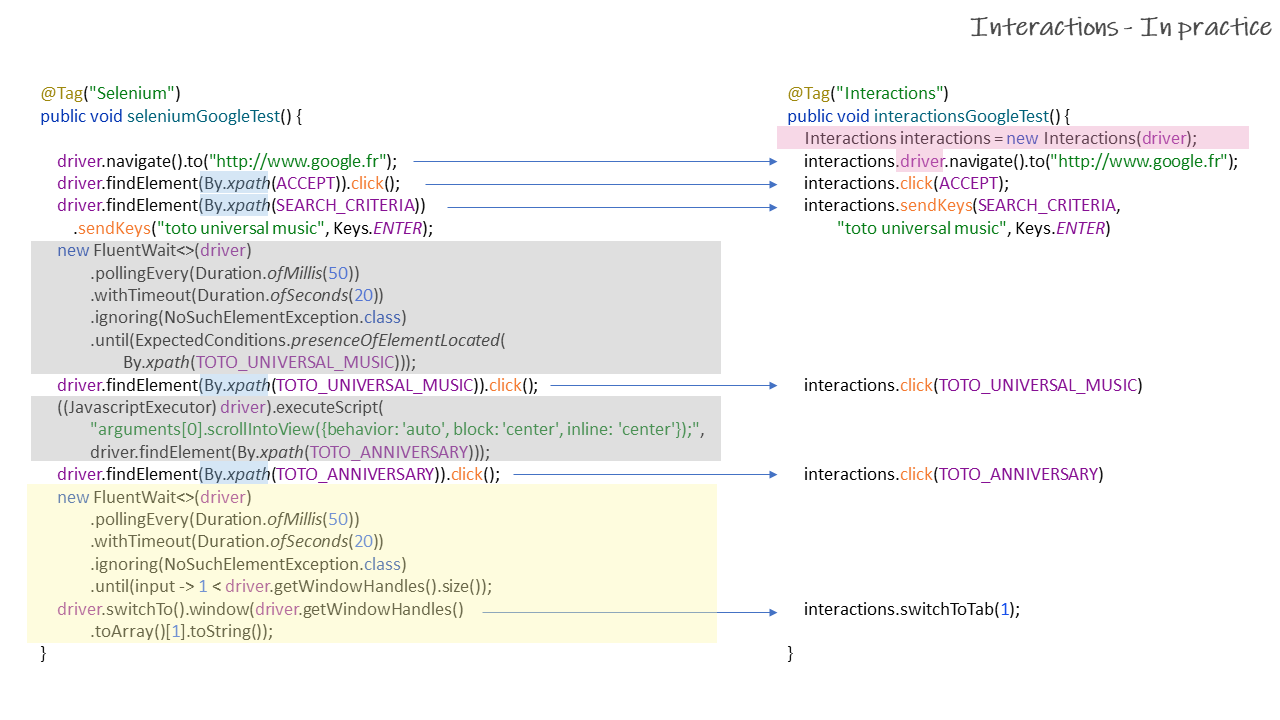
More examples available in interactions-webdriver-demo
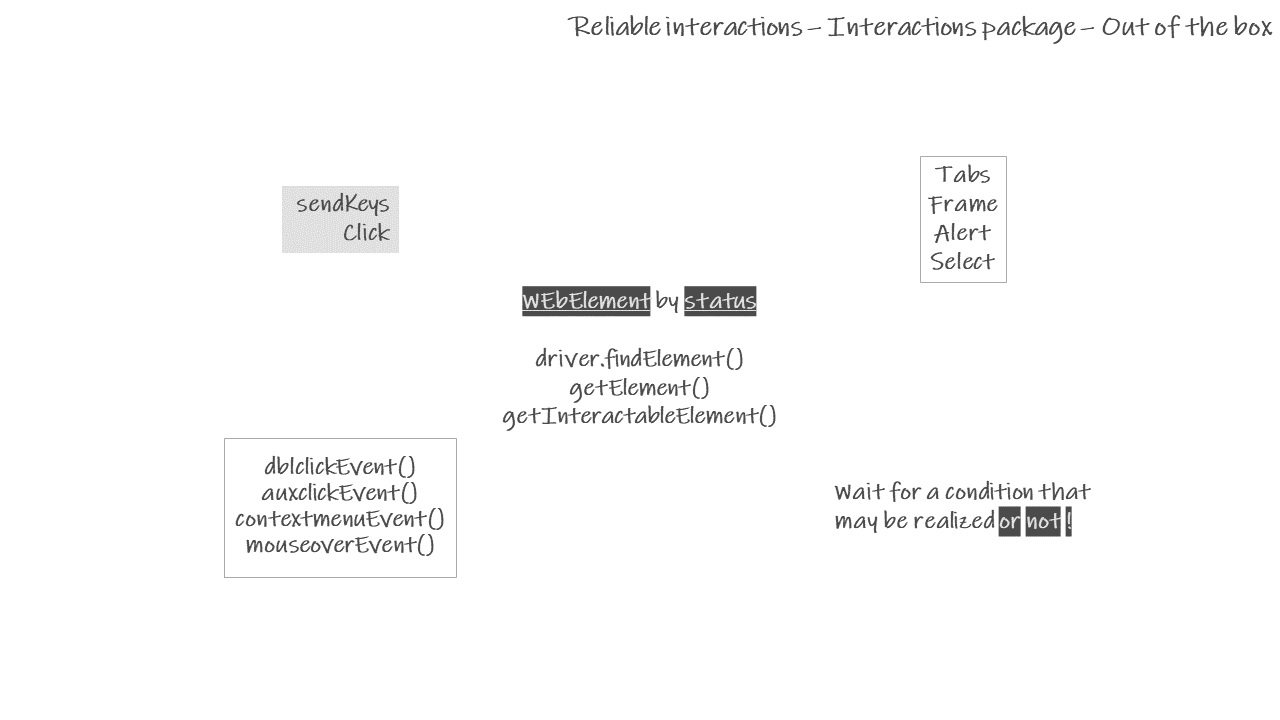
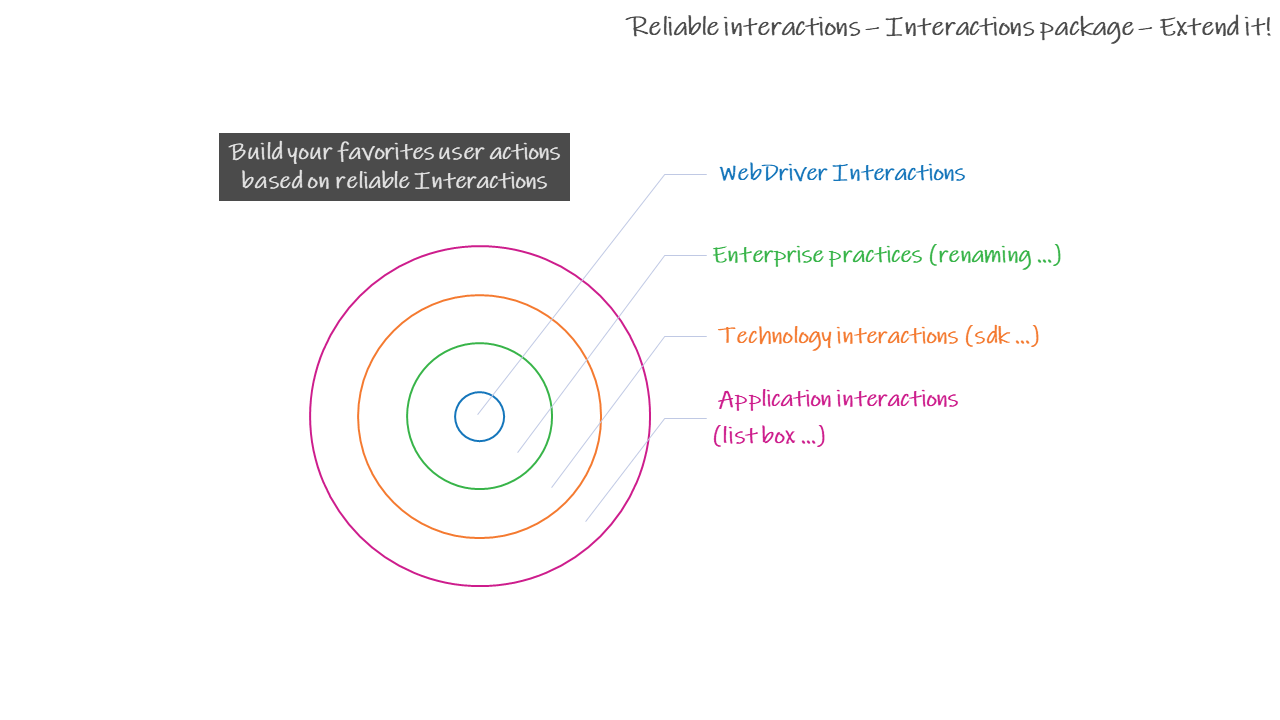
Versions
- 1.3.0 Selenium 4.1.x (compatible with 3.x)
In the box
WebDriver Interactions is based on the concept of 'ready state elements' and distinguishes 3 stages:
- unkwnown status (raw access): This stage is mainly used to check if an element exist or not
- element is present: At this stage the element can be used to access its properties
- element is interactable: At this stage the automaton can fully interact with the element (click, sendeys, ...)
WebDriver Interactions compiles a set of functions you can easely integrate in your Selenium WebDriver framework to manage technical stuff automaticaly
Usage
1. Add dependency on your pom.xml file<dependency>
<groupId>io.github.simple4tests</groupId>
<artifactId>interactions-webdriver</artifactId>
<version>...</version>
</dependency>
WebDriver driver;
...
driver = new ChromeDriver();
...
WebDriverInteractions wdi = new WebDriverInteractions(driver);
wdi.click(by);
wdi.sendKeys(by,value);
wdi. ...
wdi.driver.navivate().to(myUrl);
wdi.driver.getCurrentUrl();
wdi.driver.findElement(by);
wdi.driver. ...
Recommended implementation
Even WebDriverInteractions object can be used straight forward as shown previuosly, the recommendation is to create in your project a class Wdi that extends WebdriverInteractions. This way, you will be able to easely customize WebDriverInteractions creating your custom technical actions based on WebDriverInteractions.
public class MyWebDriverInteractions extends WebDriverInteractions {
public MyWebDriverInteractions(WebDriver driver) { super(driver); }
// custom technical action to select a value within a custom dropdown
public void selectInList(By by, String value) {... doSomething(); ...}
}
Then instanciate your newly class Wdi and you are ready to play with WebDriverInteractions functions as well as your custom functions. All ot them from a single object.
WebDriver driver;
...
driver = new ChromeDriver();
...
MyWebDriverInteractions wdi = new MyWebDriverInteractions(driver);
wdi.driver.navivate().to(url);
wdi.click(by);
wdi.sendKeys(by,value);
wdi.selectInList(by,value);
...
Objects
Notice that main of the function are already knowned by selenium expert. We try, as much as possible, to keep same name and parameters as native selenium in order to reduce the awareness effort.
We include in the documentation the main selenium function embeded in the WebDriver Interactions function.
Name | Description |
---|---|
WebDriverInteractions |
Includes functions to interact with the elements in a page as well as the following objects
|
WebDriver | The WebDriver itself used for direct calls to WebDriver functions |
JavascriptExecutor | A JavascriptExecutor object to execute javascript |
Wait | Dynamic wait engine based on a FluentWait with fancy configurations |
WebDriverInteractions functions
Constructor
Name | Description |
---|---|
WebDriverInteractions( WebDriver driver) |
WebDriverInteractions constructor initializating a Browser, a Wait and a JavaScript objects with defaut settings |
Get elements or element information
Name | Description |
---|---|
getElement(By by) | Once present, return a WebElement matching the locator |
getInteractableElement(By by) |
Once interactable, return a WebElement matching the locator. Same as getInteractableElement(by, false, true, true) |
getInteractableElement( By by, boolean waitUntilElementIsDisplayed, boolean waitUntilElementIsEnabled, boolean scrollIntoView) |
Once interactable, return a WebElement matching the locator |
count(By by) | Return the number of elements matching the locator |
isPresent(By by) | Return true is there are one or more elements matching the locator |
isAbsent(By by) | Return true is there are 0 elements matching the locator |
Interact with elements
Name | Description |
---|---|
click(By by) |
Once interactable, click on the element matching the locator Selenium : WebElement.click() |
dblclick(By by) |
Once interactable, double click on the element matching the locator Selenium : n/a |
clearAll(boolean alwaysClear) |
If true, sendKeys() will always clear the element before setting the new value. Default is false (do not clear before setting the value). |
clearNext(boolean alwaysClear) |
This function configure the next call to sendKeys() to clear or not the element before setting the new value. Return a WebDriverInteractions object
|
clear(By by) | Once interactable, clear input and textarea element matching the locator |
sendKeys( By by, CharSequence... value) |
Once interactable, set the value of the (input) element Selenium : WebElement.sendkeys() |
upload( By by, String fileAbsolutePath) |
Once interactable, upload a file using an input element Selenium : WebElement.sendkeys() |
setSelected(By by, Boolean value) |
Once interactable, set the property 'selected' of an (checkbox, radio btn) element Selenium : WebElement.click() |
Select getSelect(By by) selectByVisibleText( By by, String visibleText) selectByValue( By by, String value) selectByIndex( By by, int index) |
Once interactable, return a Select or perform the corresponding action Selenium : new Select(by) or new Select(by).xxx |
Others functions
Name | Description |
---|---|
getAlert() | One present, return the alert element |
switchToDefaultContent() |
Switch to the default content (top of the page) of the current page Selenium : driver.switchTo().defaultContent() |
switchToParentFrame() |
Switch to the parent frame of the current frame Selenium : driver.switchTo().parentFrame(); |
switchToFrame(By by) | Once available, switch to the frame identified by its locator |
switchToFrame(int index) | Once available, switch to the frame identified by its index |
switchToFrame(String nameOrId) | Once available, switch to the frame identified by its name or ID |
switchToTab(int index) |
Once present, show tab with the given index Selenium : driver.switchTo().window() |
closeTab() |
Close current tab Selenium : driver.close() |
setScrollIntoViewOptions( String behavior, String block, String inline) |
Set scrollIntoView javascript command parameters Default values :
|
scrollIntoView( WebElement webElement) |
Scroll the page to display the element as specified by the scrollIntoView's options. By default, the element is displayed in the middle of the page. |
scrollIntoView( WebElement webElement, String behavior, String block, String inline) |
Call the scrollIntoView function with custom settings |
Wait functions
Name | Description |
---|---|
Wait(WebDriver driver) | Public constructor |
pollingEvery(Duration interval) |
Set the interval to be used while performing the checks public static final Duration DEFAULT_INTERVAL = Duration.ofMillis(50) |
withTimeout(Duration timeout) |
Set the timeout to be used to perform the checks public static final Duration DEFAULT_TIMEOUT = Duration.ofMillis(10000); |
ignoreAll(Collection exceptions) | List exceptions to be ignored when performing the checks |
ignoreTimeoutException() |
By default the engine wait for a condition that must be realized. If not, there is a failure and the test stops with failed status. Some times we need to wait for a condition that may be realized (or not). This function configure the wait engine to wait for a condition to be realized and if not relalized to continue the test. Return a Wait object
|
until(Function expectedCondition) |
This functions wait for the expectedCondition to be relazided and return the result of the expectedCondition
|
until( Function expectedCondition, final Duration interval, final Duration timeout) until( Function expectedCondition, final Collection ignoredExceptions) until( Function expectedCondition, final Duration interval, final Duration timeout, final Collection ignoredExceptions) |
Wait for the expectedCondition with specific settings and return the result of the expectedCondition |
static until( Function expectedCondition, WebDriver driver, Duration interval, Duration timeout, Collection ignoredExceptions) |
Wait for the expectedCondition with custom settings and return the result of the expectedCondition |